Spring Framework is one of the most popular Java frameworks for building enterprise applications.
At the heart of Spring lies the concept of beans, which are the foundation of Spring’s dependency injection (DI) system.
But what exactly are beans in Spring? How do they work? Let’s dive in and break it down in a simple, beginner-friendly way.
What Is a Bean in Spring?
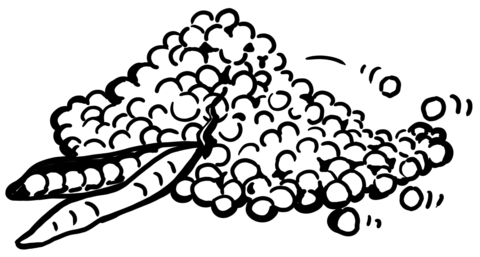
In Spring, a bean is an instance of a class, same as any instance you create by yourself in java
class Test {
private int i;
}
public static void main(String[] args) {
Test t = new Test();
}
But the key difference is its instantiated, assembled, and managed by the framework and put into a "bag of objects" (the container) from where you can get them later.
In a simple words, beans are Java objects that Spring handles for you, ensuring proper dependency injection and lifecycle management.
How Are Beans Defined in Spring?
There are many ways to define beans in Spring
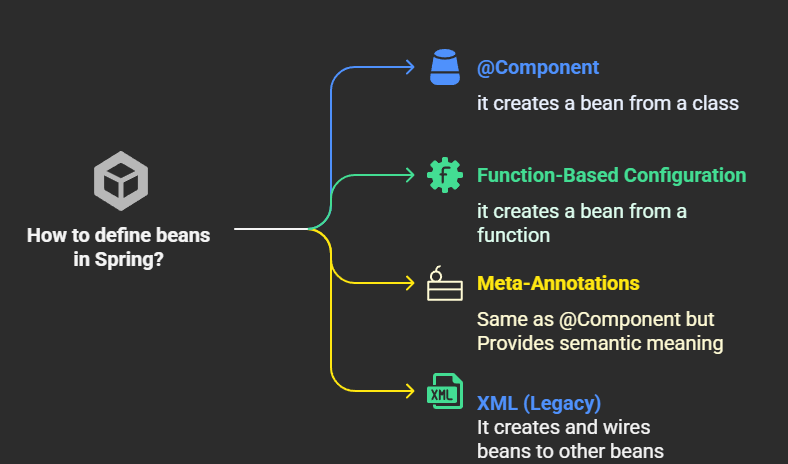
1. Using @Component (General Bean Definition)
The @Component
annotation is a generic way to mark a class as a Spring bean. Spring automatically detects and registers it when component scanning is enabled.
import org.springframework.stereotype.Component;
@Component
public class MyComponentBean {
public void doSomething() {
System.out.println("MyComponentBean is working!");
}
}
2. Using Function-Based Configuration
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AppConfig {
@Bean
public MyBean myBean() {
return new MyBean();
}
}
3. Meta-Annotations
All the other annotations (@Service
, @Repository
, @Controller
, @RestController
and many other ) are called Meta Annotation, because they derive from @Component
These annotation serve the same technical function—registering beans in the Spring IoC container. However, they add semantic meaning to the code, making it clear which layer a class belongs to which enhance code readability .
4.XML configuration(legacy)
Before Java-based configuration and annotations became the standard, XML configuration was the primary way to define beans in Spring.
Developers would declare beans inside an XML file, specifying their class, dependencies, and lifecycle methods.
Example of XML Bean Definition
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="myBean" class="com.example.MyBean"/>
</beans>
Final Thoughts
Spring beans are the backbone of any Spring application, handling dependencies and lifecycle management seamlessly.
Whether you define beans using XML, Java configuration, or annotations, they play a crucial role in building scalable, maintainable applications.
Want to learn more?
Want to dive deeper into Spring? Start by experimenting with different bean scopes and lifecycle methods in your projects!
👉 Read Next: What Is Dependency Injection In Spring?