Spring is one of the most popular Java frameworks, known for its powerful features that simplify Java development. One of its core principles is Dependency Injection (DI)—a design pattern that makes applications more modular, testable, and maintainable.
But what exactly is Dependency Injection, and why is it so crucial in Spring?
Let’s dive in and explore its concepts, types, and practical implementation with code examples.
What is Dependency Injection (DI)?
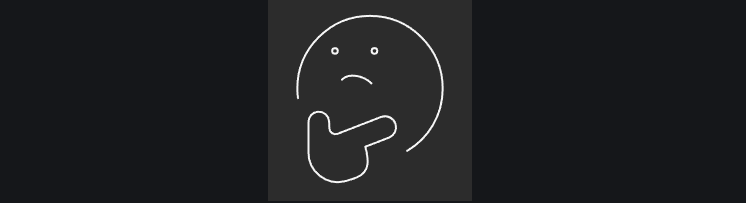
Dependency Injection is a design pattern used in Spring’s Inversion of Control (IoC) container to manage object dependencies.
Instead of an object creating its dependencies, DI allows these dependencies to be injected by an external container.
How Did It Start?
Before DI, developers manually created dependencies within a class, making code tightly coupled and hard to maintain.
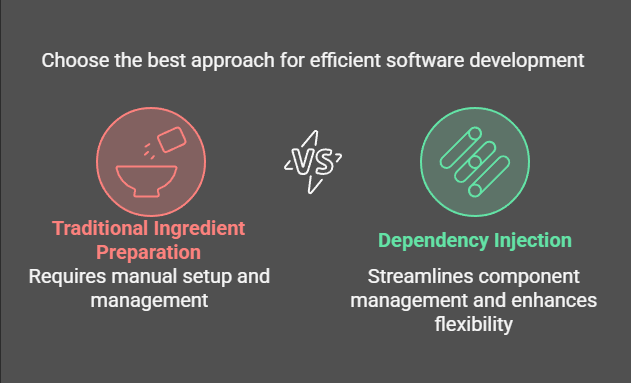
Then came Inversion of Control (IoC), allowing external frameworks to handle object creation and dependency management
1.Before Dependency Injection:
class Car {
private Engine engine;
// Manually creating dependency
public Car() {
this.engine = new Engine();
}
}
2.After Dependency Injection:
class Car {
private Engine engine;
// Injecting dependency via constructor
public Car(Engine engine) {
this.engine = engine;
}
}
With DI, Car
no longer creates Engine
but receives it externally
So How does Spring rely on DI to work
Spring relies on Dependency Injection (DI) to manage object creation and dependencies efficiently within Spring Container. Here's how:
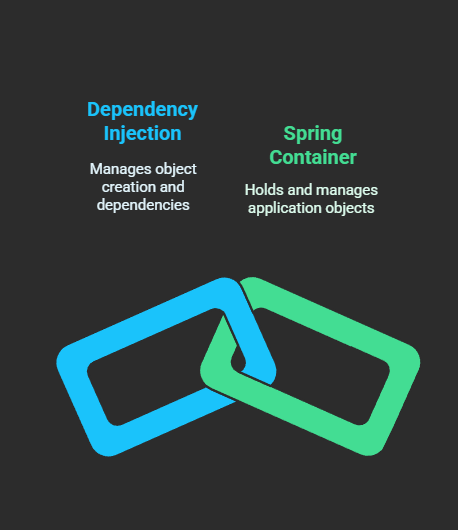
- Object Creation: Spring automatically creates and manages objects instead of developers instantiating them manually.
- Spring Context: Spring stores these objects in Spring Context.
- Centralized Control: The Spring ensures that dependencies are managed centrally in Spring Context, reducing tight coupling and improving maintainability.
- Lifecycle Handling: Spring controls the lifecycle of objects, initializing, injecting dependencies, and destroying them when no longer needed.
What Are the Different Types of Injection?
Spring provides multiple ways to inject dependencies:
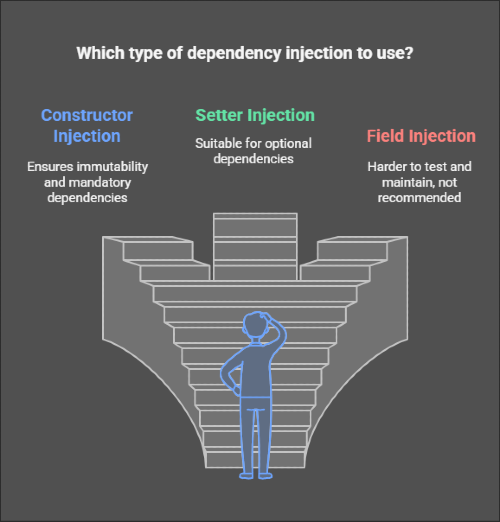
1.Constructor Injection (Recommended)
- Dependencies are injected via the constructor.
- Ensures immutability and mandatory dependencies.
- Example:
public class Car {
private final Engine engine;
@Autowired
public Car(Engine engine) {
this.engine = engine;
}
}
2.Setter Injection
- Uses setter methods to inject dependencies.
- Useful when dependencies are optional.
- Example:
@Component
public class Car {
private Engine engine;
@Autowired
public void setEngine(Engine engine) {
this.engine = engine;
}
}
3.Field Injection (Not Recommended)
- Uses @Autowired directly on fields.
- Harder to test and maintain.
- Example:
@Component
public class Car {
@Autowired
private Engine engine;
}
Conclusion
Dependency Injection is at the heart of the Spring Framework, making applications more modular, scalable, and easier to test.
Whether you use Constructor Injection, Setter Injection, or Field Injection, understanding these techniques will help you build efficient Spring applications.
Want to learn more?
👉 Read Next: What are Beans in Spring?