In the world of Java development, Spring has become synonymous with enterprise applications. However, newcomers to the ecosystem often find themselves confused by the terminology: Spring Framework, Spring Boot, Spring MVC, Spring Cloud, and so on.
This article aims to clarify the most fundamental distinction: the difference between Spring Framework and Spring Boot.
What is Spring Framework?
Spring Framework is the original foundation of the entire Spring ecosystem. Introduced in 2004, it was created to address the complexity of enterprise application development.
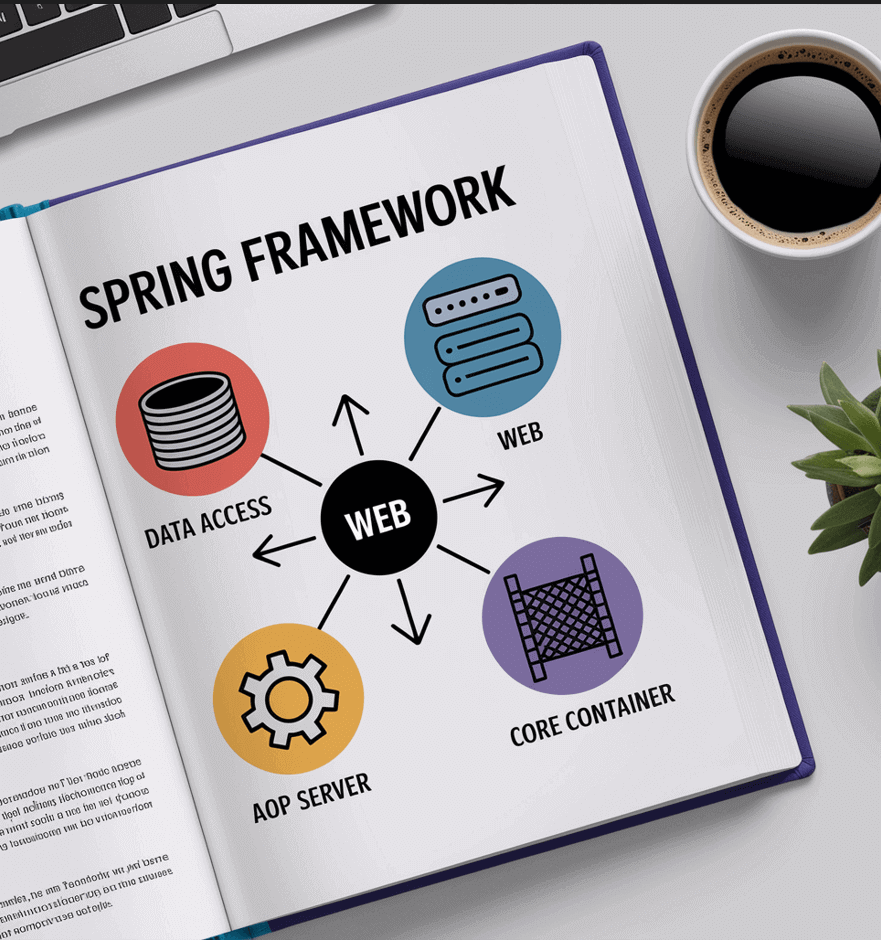
The Challenge with Spring Framework
While powerful, Spring Framework requires significant configuration and setup. Traditionally, XML configuration files were necessary, which could become verbose and difficult to maintain.
Later versions introduced annotation-based and Java-based configurations, but setting up a project still required numerous decisions and boilerplate code.
What is Spring Boot?
Spring Boot, launched in 2014, is not a replacement for Spring Framework but rather a solution to the configuration complexity. Think of it as an extension that makes working with Spring Framework significantly easier.
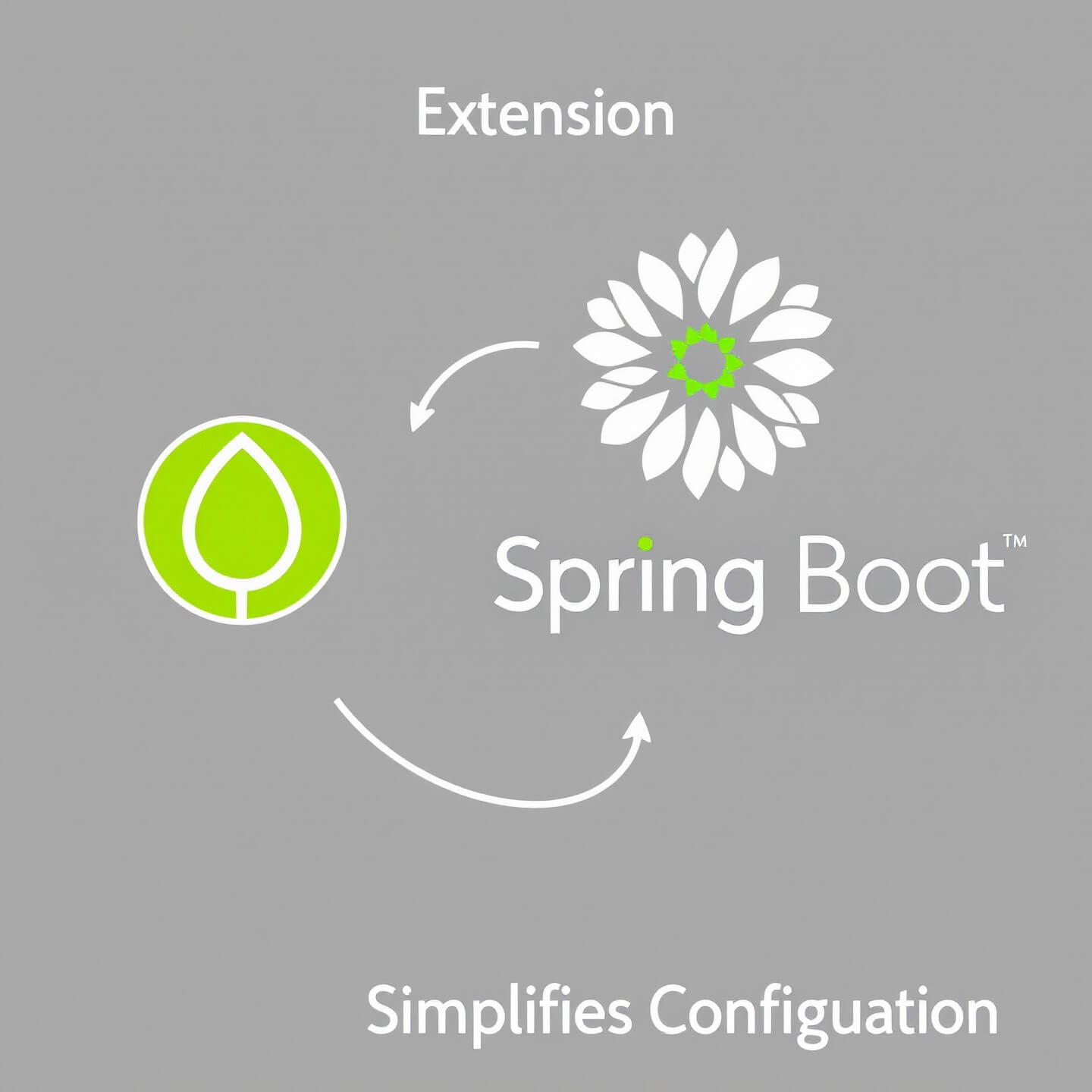
How Spring Boot Simplifies Development, Consider a simple web application.
With Spring Framework alone
You would need to:
- Configure a Dispatcher Servlet
public class MyWebAppInitializer extends AbstractAnnotationConfigDispatcherServletInitializer {
@Override
protected Class<?>[] getRootConfigClasses() {
return new Class<?>[] { RootConfig.class };
}
@Override
protected Class<?>[] getServletConfigClasses() {
return new Class<?>[] { WebConfig.class };
}
@Override
protected String[] getServletMappings() {
return new String[] { "/" };
}
@Override
protected void customizeRegistration(ServletRegistration.Dynamic registration) {
// Optional: custom settings for the DispatcherServlet
registration.setInitParameter("throwExceptionIfNoHandlerFound", "true");
}
}
- Set up view resolvers
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Bean
public ViewResolver viewResolver() {
InternalResourceViewResolver resolver = new InternalResourceViewResolver();
resolver.setPrefix("/WEB-INF/views/");
resolver.setSuffix(".jsp");
resolver.setViewClass(JstlView.class);
resolver.setExposeContextBeansAsAttributes(true);
return resolver;
}
}
- Configure a web server
public class WebAppInitializer implements WebApplicationInitializer {
@Override
public void onStartup(ServletContext servletContext) throws ServletException {
// Configure Spring context and DispatcherServlet
// ...
}
}
With Spring Boot
you can achieve the same with minimal configuration:
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
The difference in complexity is stark!
The Relationship: Spring Boot is Built on Spring Framework
A critical point to understand is that Spring Boot is built on top of Spring Framework—it's not an alternative to it. When you use Spring Boot, you're still using Spring Framework under the hood.
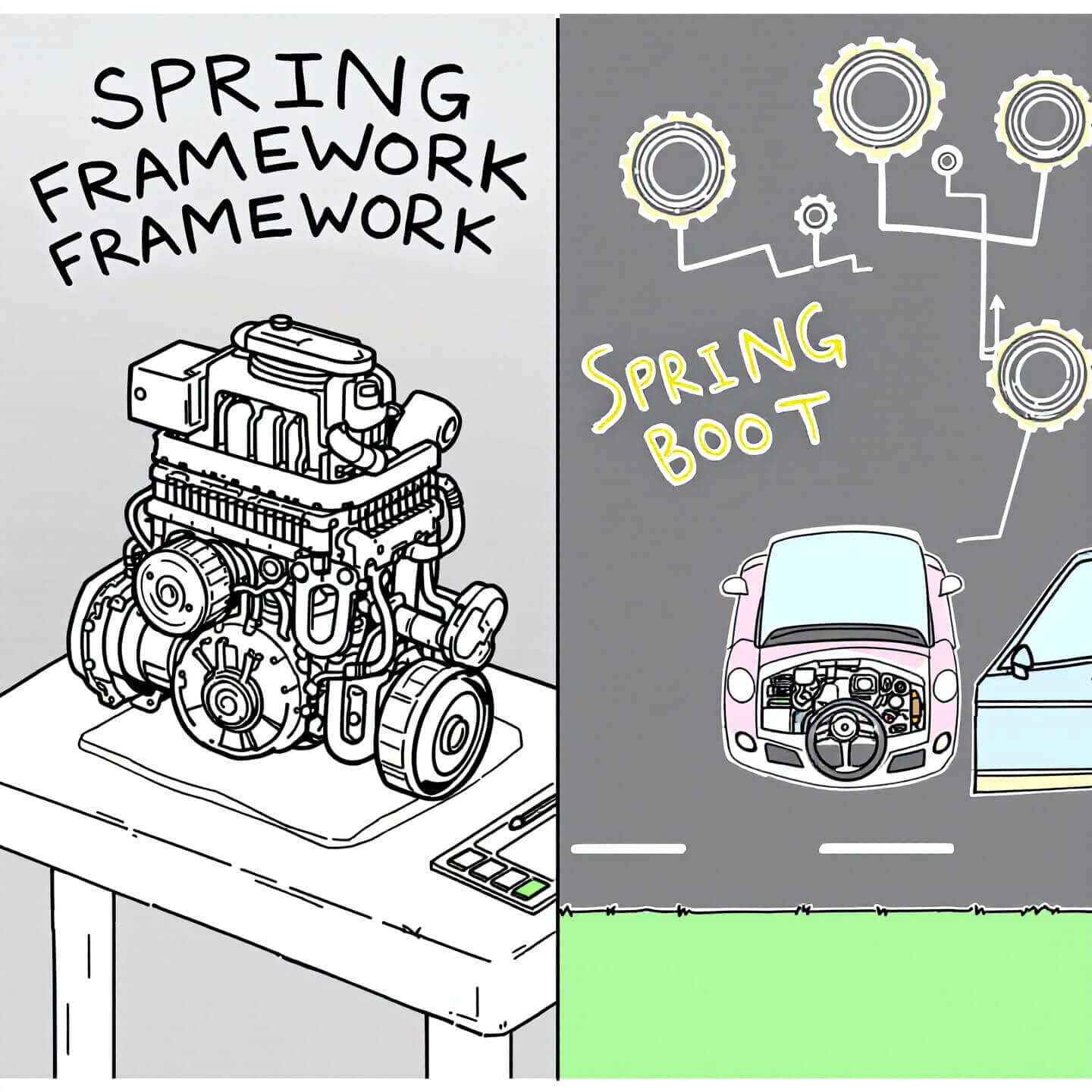
Think of their relationship as:
- Spring Framework: The engine and core components
- Spring Boot: A streamlined interface to interact with that engine
When to Use Spring Framework vs Spring Boot
Use Spring Framework When:
- You need precise control over every aspect of the configuration
- You're working with legacy systems that have existing Spring setups
- You require only specific components of the Spring ecosystem
- You have constraints that conflict with Spring Boot's opinions.
- Example: Banks frequently mandate specific legacy systems, older database versions, or proprietary middleware that don't align well with Spring Boot's modern defaults
Use Spring Boot When:
- You're starting a new project and want rapid development
- You prefer convention over configuration
- You need to deploy standalone applications
- You want production-ready features out-of-the-box
- You don't have specific requirements that conflict with Spring Boot's defaults
Common Misconceptions
there are so many misconceptions about Spring Boot, here are some:
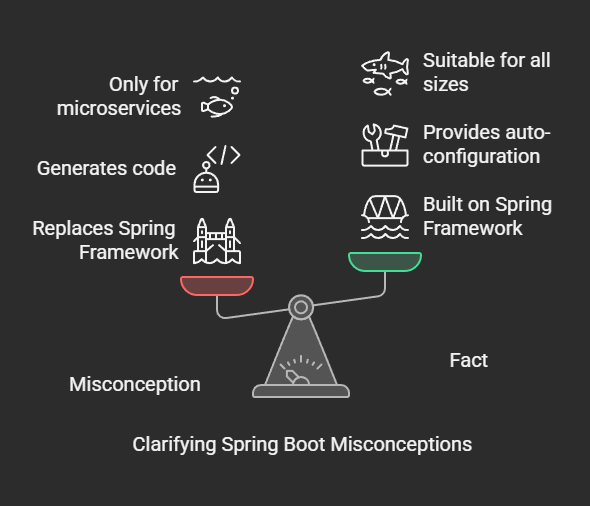
- "Spring Boot replaces Spring Framework": False. Spring Boot is built on Spring Framework.
- "Spring Boot generates code": False. It provides auto-configuration but doesn't generate code.
- "Spring Boot is only for microservices": False. While excellent for microservices, Spring Boot is suitable for applications of any size.
- "Spring Boot is less flexible": Partially true. While it imposes defaults, you can override virtually any configuration.
Conclusion
The choice between Spring Framework and Spring Boot isn't really a choice at all for most new applications. Spring Boot provides significant advantages in development speed and simplicity without sacrificing the power of Spring Framework.
For new projects, Spring Boot is almost always the recommended starting point. As you become more familiar with the ecosystem, you'll appreciate how Spring Boot removes much of the boilerplate while still allowing you to leverage the full capabilities of Spring Framework when needed.
Remember that Spring Boot is Spring Framework—just with a lot of the configuration headaches removed.
Want to learn more?
👉 Read Next: What is IoC container and how Spring use it
👉 Read Next: What Is Dependency Injection In Spring
👉 Read Next: What are Beans in Spring?