If you've ever worked with Java applications, you know managing dependencies can become complex as the project grows.
That's where Inversion of Control (IoC) comes in. IoC is a design principle that helps manage object creation and dependencies efficiently.
Spring Framework is one of the most popular frameworks that leverages IoC to simplify Java application development. But how does it work? Let's explore.
The Problem Before IoC
Before IoC, developers had to manually create and manage object dependencies. This meant:
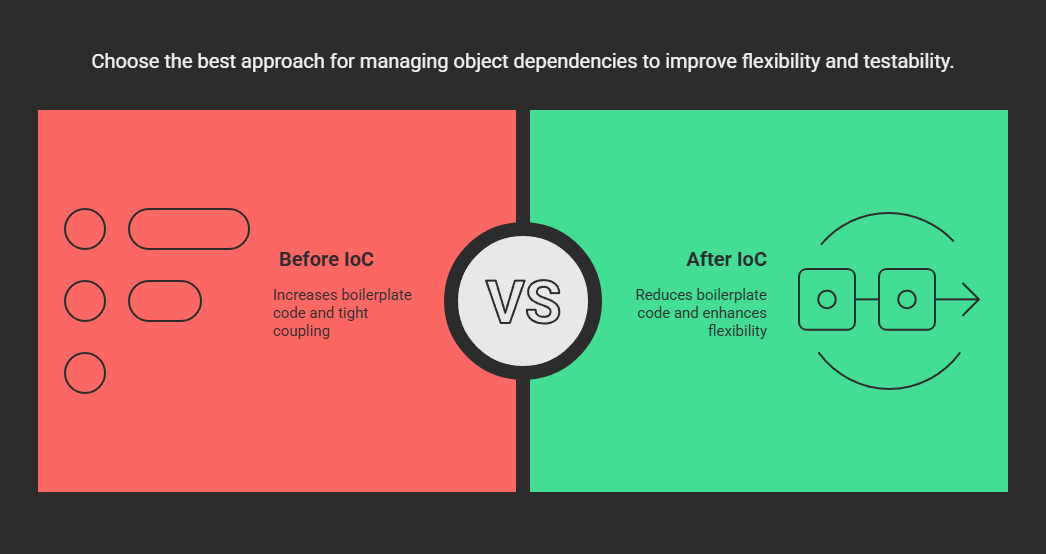
- Writing a lot of boilerplate code to instantiate objects.
- Hardcoded dependencies, making it difficult to change implementations.
- Tight coupling between classes, reducing flexibility and making testing harder.
For example, imagine a Car class that depends on an Engine class:
public class Car {
private Engine engine;
public Car() {
this.engine = new Engine(); // Hardcoded dependency
}
}
If we wanted to switch to a different Engine implementation, we would have to modify the Car class, making maintenance and testing harder.
So What Is IoC?
IoC is a principle where the control of object creation and dependency management is inverted to a container or framework instead of being manually handled in the code.
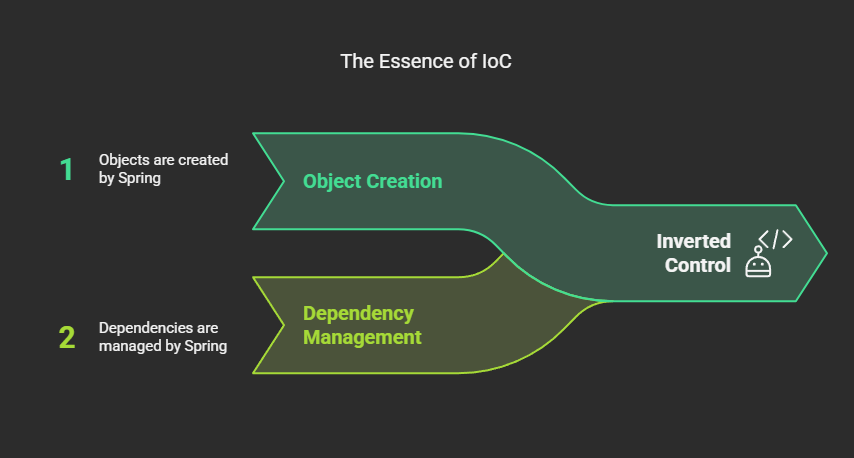
This means that instead of creating objects inside your classes, you delegate this responsibility to an IoC container. In Spring, this container is called the Spring IoC Container.
How Spring Uses IoC
Spring Framework implements IoC through Dependency Injection (DI). There are multiple ways to inject dependencies in Spring, but here is one of them (constructor injection). Here’s how it works:
@Component
public class Engine {
public void start() {
System.out.println("Engine started");
}
}
@Component
public class Car {
private Engine engine;
@Autowired // Spring initiates Engine first and injects it here
public Car(Engine engine) {
this.engine = engine;
}
}
Pros vs Cons of IoC in Spring
Spring, like everything, has its downsides and upsides. For example:
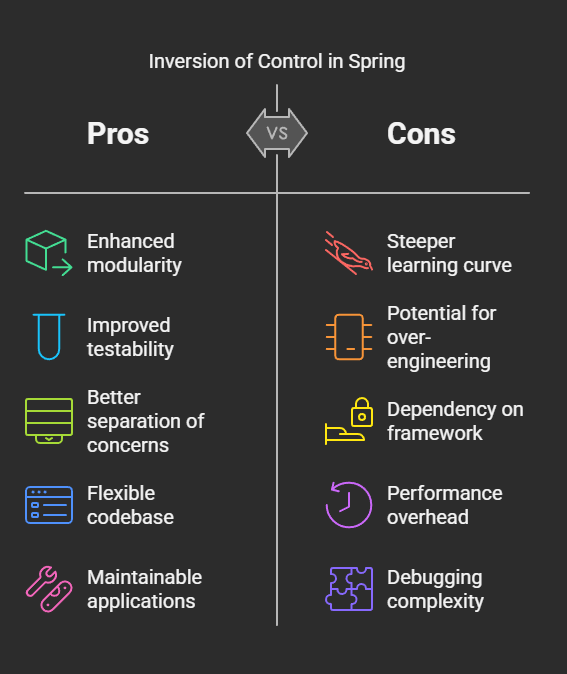
1.Advantages of IoC in Spring
- Loose Coupling: Reduces dependency between classes, making them easier to manage and test.
- Better Maintainability: Changes in dependencies don’t require modifications in dependent classes.
- Easier Testing: With dependencies injected, mocking becomes easier for unit testing.
2.Disadvantages of IoC
- Learning Curve: Beginners may find it challenging to understand dependency injection initially.
- Slight Performance Overhead: The IoC container consumes memory and processing power.
Conclusion
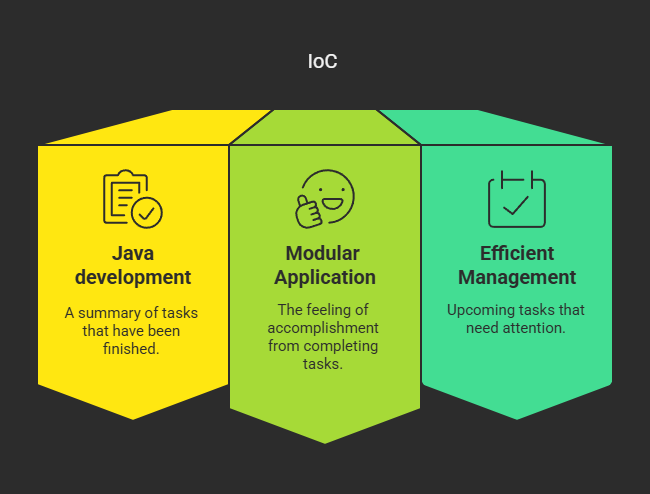
IoC is a game-changer in Java development, making applications more modular, testable, and maintainable. Spring’s IoC container efficiently manages dependencies using Dependency Injection, simplifying the way we build Java applications.
Want to learn more?
👉 Read Next: What Is Dependency Injection In Spring
👉 Read Next: What are Beans in Spring?
👉 Read Next: What Is Bean Lifecycle In Spring