Hibernate has introduced the Persistence Context to manage the entity lifecycle within an application.
In this blog, we’ll first introduce what is Persistence Context and highlight its importance. then we will go through its use cases.
What is Persistence Context?
The persistence context is a temporary storage area (cache) that sits between your application and the database. It keeps track of entities that are loaded from or saved to the database.
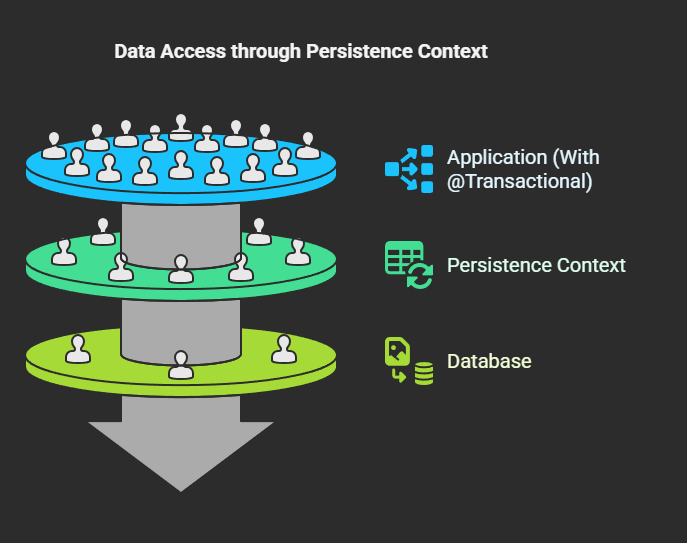
When an entity is loaded, the persistence context manages it. If you make changes to this entity during a transaction, the persistence context marks it as changed (or "dirty"). At the end of the transaction, all changes are saved to the database in one go.
If every small change in an entity went straight to the database, it would require many database calls. Database calls are slow and expensive, so this approach would hurt performance. The persistence context reduces this by grouping multiple changes into fewer database calls.
How Do We Use Persistence Context
The EntityManager
is how your application communicates with the persistence context. When you use the EntityManager
, you're working directly with the persistence context.
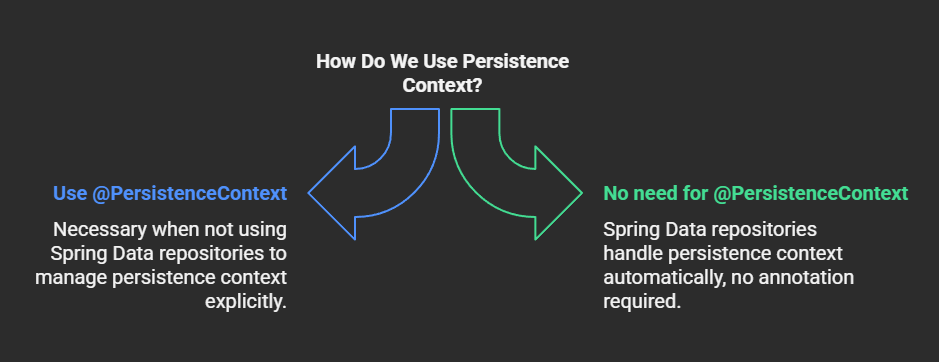
So, we need to tell the EntityManager
to use this type, we use the annotation @PersistenceContext
like this:
@PersistenceContext
private EntityManager entityManager;
If you are using Spring Data repositories then no need to explicitly write @PersistenceContext
because it handles this internally as it is the default type.
Persistence Context Example
We will go with Spring Data Repository example:
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional
public void fetchUserTwice(Long id) {
// FIRST FETCH – from database
User user1 = userRepository.findById(id).orElse(null);
// SECOND FETCH – from persistence context (cached)
User user2 = userRepository.findById(id).orElse(null);
}
}
Conclusion
In this Blog, we have learned the concept of Persistence Context in Hibernate.
First, we defined what is persistence context, then we walked through how it works demonstrating its behavior with a real-life example to simplify understanding.
Want to learn more?
- 📩 Subscribe
👉 Read Next: What Are Types of Persistence Contexts?
👉 Read Next: What are Beans in Spring?
👉 Read Next: What Is Bean Lifecycle In Spring