The Spring Context is crucial because it provides an environment where objects (or beans) are created, managed, and assembled. This management makes your code easier to test, maintain, and scale.
For beginners, grasping this concept can hard specially there is a lot of the magic behind dependency injection and inversion of control in Spring.
The Problem Before Spring Context
Before the Spring Container was introduced developers had to write extensive code to instantiate objects, handle dependencies, and manage their lifecycle.
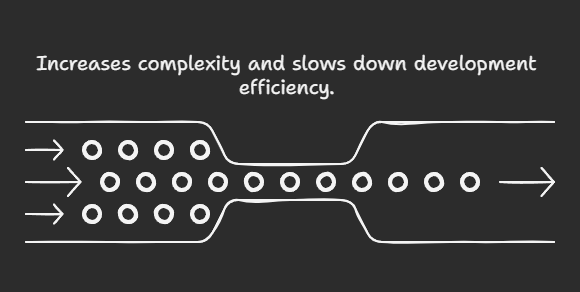
This process was time consuming and error-prone. The lack of a centralized management system led to tightly coupled code, making maintenance a challenge.
What Is Spring Context (Spring Container)?
And here Spring comes and creates it for you, instead of manually creating objects using new
, and keeps them inside the container. Later, when you need an object, Spring fetches it from the container and injects it where required.
Imagine a box where Spring stores all the objects (beans) it creates. This box is called the Spring Context (Spring Container).
Types of Spring Contexts
Spring has 2 types of context implementations:
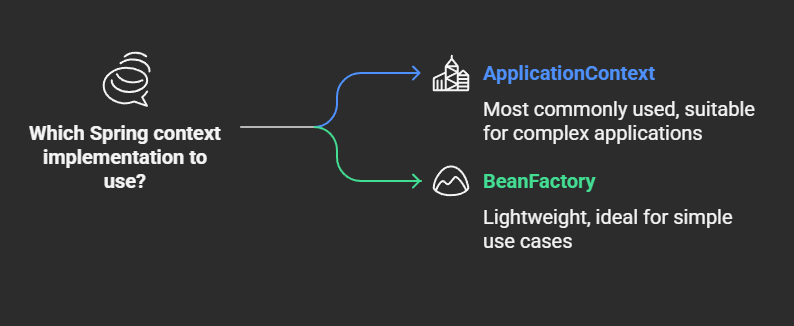
- ApplicationContext (Most commonly used)
public static void main(String[] args) {
ApplicationContext ctx = new AnnotationConfigApplicationContext(cfg.class);
MyService service = ctx.getBean(MyService.class);
service.someRandomgFunction();
}
- BeanFactory (Lightweight, used for simple use cases)
public static void main(String[] args) {
DefaultListableBeanFactory bf = new DefaultListableBeanFactory();
AnnotatedBeanDefinitionReader r = new AnnotatedBeanDefinitionReader(bf);
r.register(MyService.class); // Registering annotated bean
MyService service = beanFactory.getBean(MyService.class);
service.someRandomgFunction();
}
⚠️PS: Spring Context (Spring Container) isn’t limited to standalone applications. It is also present in Spring Web applications and other Spring-based projects.
What are Pros and Cons of Spring Context
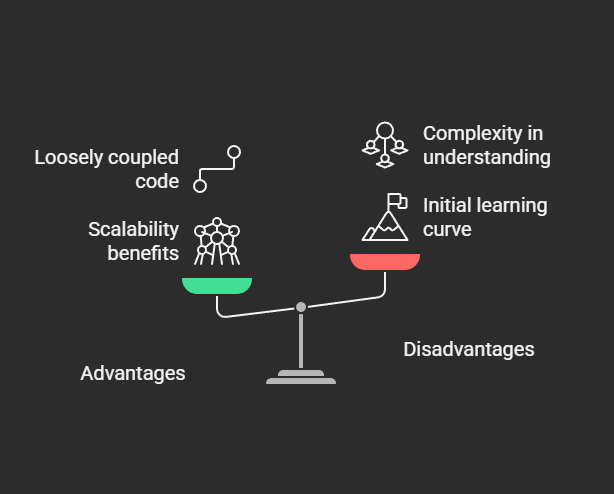
The benefits of Spring Context make it a powerful tool for managing large enterprise applications.
Advantages
- Scalability – Large applications benefit from it and that's one of the reasons why enterprises use it.
- Loosely coupled code – Objects don’t create their dependencies manually.
- Better resource management – Beans are efficiently created and managed.
Disadvantages
- Initial learning curve – Understanding IoC and DI takes time to learn and understand. (That's why we at Amdose help developers master Spring in a short, effective, and hands-on learning experience)
Want to learn more?
👉 Read Next: What Is Dependency Injection In Spring
👉 Read Next: What are Beans in Spring?
👉 Read Next: What Is Bean Lifecycle In Spring