Spring PetClinic is one of the most popular sample applications for learning the Spring Framework. it demonstrates Spring Framework best practices and serves as an educational resource for developers at all levels.
In this blog post, we'll explore what makes PetClinic valuable, how it's structured, and why you might want to use it as a learning tool.
What is Spring PetClinic?
Spring PetClinic is an open-source reference application that simulates a veterinary clinic management system. It's maintained by the Spring team and demonstrates how to build a full-featured application using the Spring ecosystem.
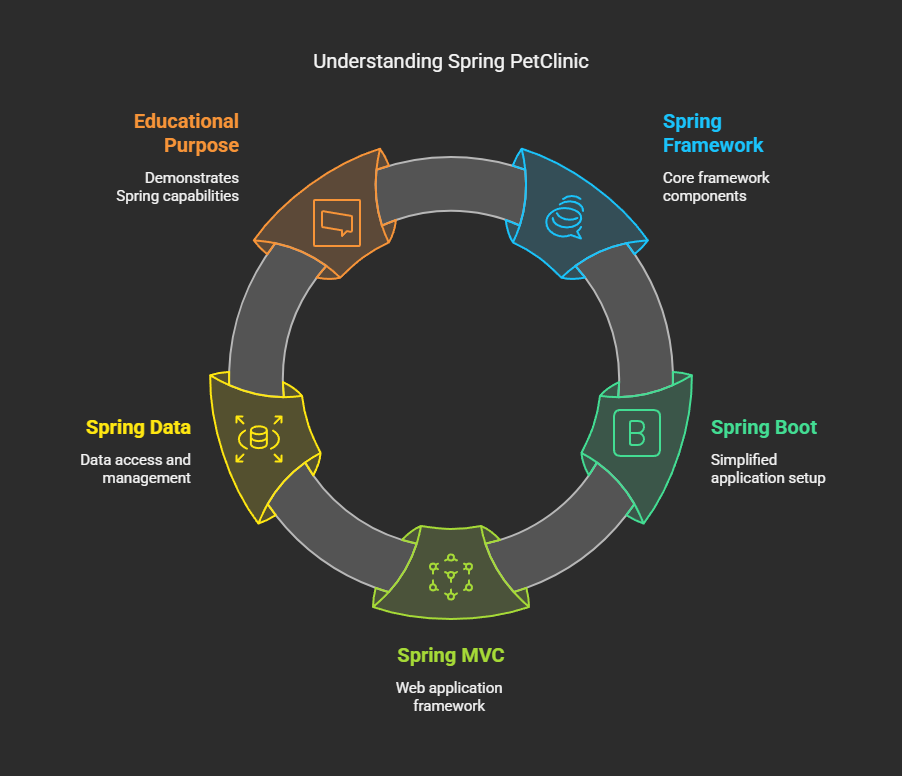
The primary goal of PetClinic isn't to provide a production-ready solution but rather to showcase Spring's capabilities and serve as a template for understanding how different Spring components work together.
Key Features and Technologies
PetClinic incorporates numerous Spring technologies and design patterns:
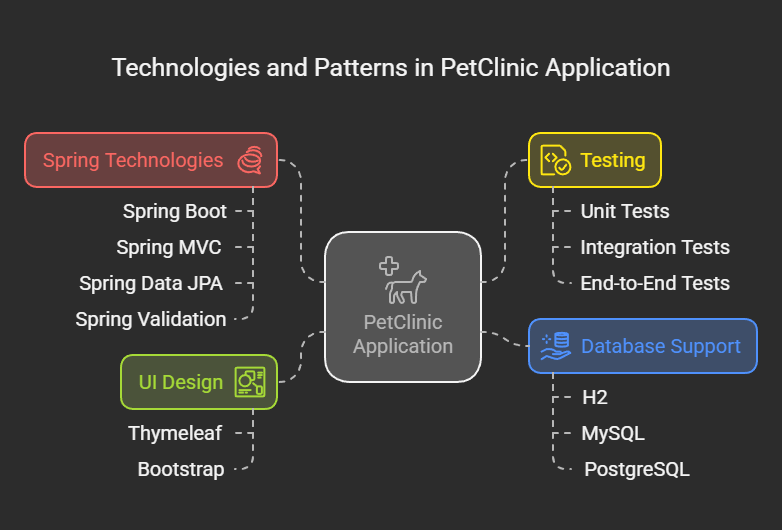
- Spring Boot: The application is built on Spring Boot, making it easy to run as a standalone application.
- Spring MVC: For handling HTTP requests and rendering views.
- Spring Data JPA: For data access and persistence.
- Thymeleaf: As the view templating engine.
- Bootstrap: For responsive UI design.
- Spring Validation: For form validation.
- Testing: Includes unit tests, integration tests, and end-to-end tests.
- Database support: Works with H2 (in-memory), MySQL, and PostgreSQL.
Project Structure
One of PetClinic's strengths is its clean architecture. The application follows a standard layered architecture:

- Presentation Layer: Controllers handle HTTP requests and delegate to services.
- Service Layer: Contains business logic and coordinates activities.
- Data Access Layer: Repositories that handle database operations.
- Domain Layer: Entity classes that represent the business domain.
This separation of concerns makes the codebase easier to understand and maintain.
Learning Opportunities
PetClinic offers several learning opportunities for developers:
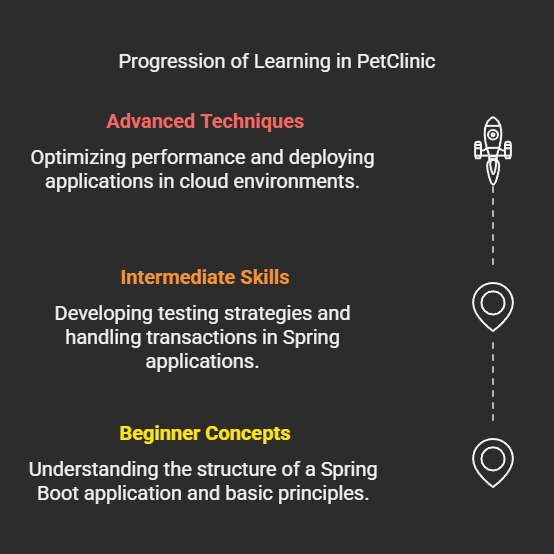
For Beginners
- Understanding a complete Spring Boot application structure
- Learning about dependency injection and IoC principles
- Seeing how controllers, services, and repositories work together
- Basics of database access with JPA
For Intermediate Developers
- Testing strategies for Spring applications
- Form handling and validation
- Transaction management
- Database migrations
- Proper exception handling
For Advanced Developers
- Performance optimizations
- Extending the application with new features
- Implementing alternative front-ends (REST API, Angular, React)
- Containerization and cloud deployment
Variants and Forks
The popularity of PetClinic has led to multiple variants that demonstrate different technologies:
- Spring PetClinic REST: A RESTful API version
- Spring PetClinic Angular: Frontend implemented with Angular
- Spring PetClinic Reactive: Using Spring WebFlux for reactive programming
- Spring PetClinic microservices: Broken down into microservices
Each variant provides insights into how Spring can be used in different architectural styles.
Getting Started with PetClinic
To start exploring PetClinic, follow these steps:
- Clone the repository:
git clone https://github.com/spring-projects/spring-petclinic.git
- Navigate to the project directory:
cd spring-petclinic
- Run the application:
./mvnw spring-boot:run
- Access the application at
http://localhost:8080
The code is well-documented, making it easy to understand how everything fits together.
Why PetClinic Matters
PetClinic's significance extends beyond being just another sample application:
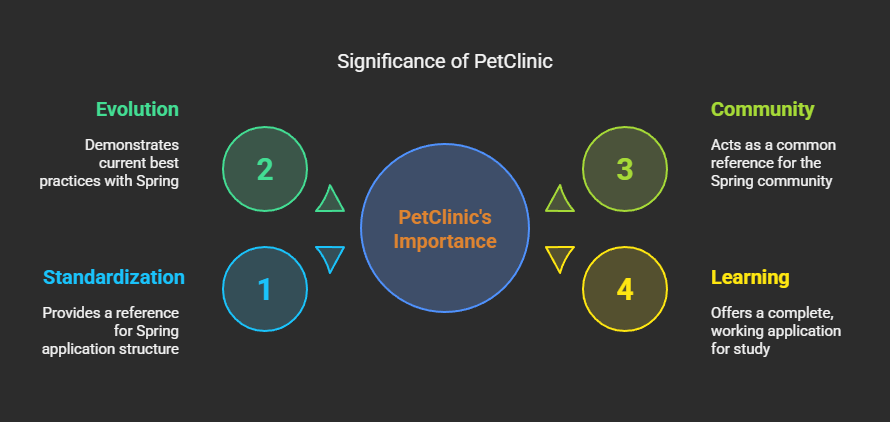
- Standardization: It provides a standard reference for how Spring applications should be structured.
- Evolution: The application evolves with Spring itself, always demonstrating current best practices.
- Community: It serves as a common reference point for the Spring community.
- Learning: It offers a complete, working application that developers can study and extend.
Conclusion
Spring PetClinic represents more than just a simple demo application—it's a valuable educational resource and a living example of Spring best practices. Whether you're new to Spring or an experienced developer looking to sharpen your skills, PetClinic offers something for everyone.
The next time you start a new Spring project, consider taking a look at PetClinic first—it might just save you time and help you avoid common pitfalls.
Want to learn more?
👉 Read Next: What Is Persistence Contexts In JPA?
👉 Read Next: What are Beans in Spring?
👉 Read Next: What Is Bean Lifecycle In Spring